Reading And Writing File In Java
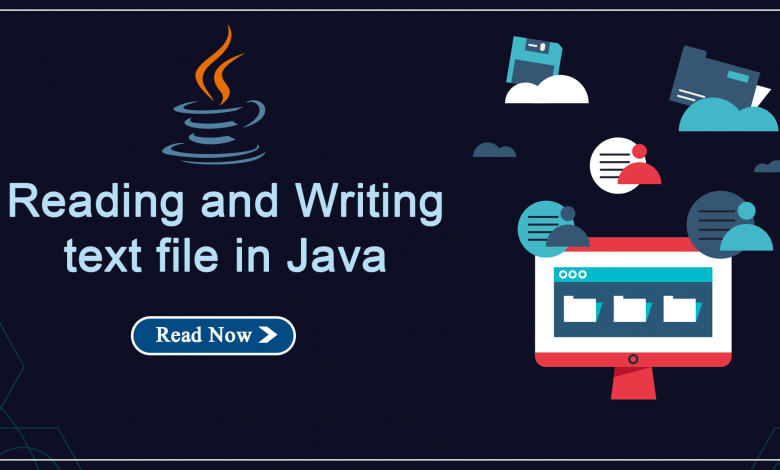
- Introduction to Reading a text File in Java:
- There are many ways to read a plain text file in Java.
- We are using FileReader, BufferedReader, and Scanner to read a text file.
- This utility provides something special like:
- BufferedReader: It provides buffering of data for fast reading.
- Scanner: It provides parsing ability.
A. BufferedReader:
- The BufferReader reads the text from a character-input stream.
- It also did buffering for the reading of the characters, arrays, and the lines.
Let's have an Example:
BufferedReader in = new BufferedReader(Reader in, int size);
Program:
import java.io.*; public class ReadFile { public static void main(String[] arg)throws Exception { File fi = new File("C:\\Users\\read\\Desktop\\test.txt"); BufferedReader br = new BufferedReader(new FileReader(fi)); String st; while ((st = br.readLine()) != null) System.out.println(st); } }
B. FileReader Class: It is a class for reading character files.
import java.io.*; public class ReadingFile { public static void main(String[] arg) throws Exception { FileReader fileread= new FileReader("C:\\Users\\test\\Desktop\\test.txt"); int i; while ((i=fileread.read()) != -1) System.out.print((char) i); } }
C. Scanner Class:
- A scanner which can parse the primitive types and also using strings through regular expressions.
- A Scanner class can break its input into tokens, which by default matches whitespace.
- These resulting tokens may then be converted into values of different types using the various next methods.
// Java Program to illustrate reading from Text File // using Scanner Class import java.io.File; import java.util.Scanner; public class ReadFromFileUsingScanner { public static void main(String[] arg) throws Exception { // pass the path to the file as a parameter File file = new File("C:\\Users\\test\\Desktop\\test.txt"); Scanner sc = new Scanner(file); while (sc.hasNextLine()) System.out.println(sc.nextLine()); } }
2. Introduction to Writing a text File in Java:
a. Java BufferedWriter Class:
- Java BufferedWriter class is used to provide buffering for Writer instances.
- It makes the performance fast.
- It inherits the Writer class.
- The buffering characters are used for delivering the efficient writing of single arrays, characters, and strings.
- Creates a buffered character-output stream that uses a default-sized output buffer.
public class BufferedWriter extends Writer
Program:
import java.io.*; public class NewClass { public static void main(String[] args) { //initializing FileWriter FileWriter filewrite; try { filewrite = new FileWriter("ABC.txt"); // Initialing BufferedWriter BufferedWriter bufferwrite = new BufferedWriter(filewrite); System.out.println("Buffered Writer start writing :)"); // Use of write() method to write the value in 'ABC' file bufferwrite.write(69); bufferwrite.write(49); // Closing BufferWriter to end operation bufferwrite.close(); System.out.println("Written successfully"); } catch (IOException excpt) { excpt.printStackTrace(); } } }
b) Java FileWriter Class
- This class is used to write character-oriented data to a file.
- It is a character-oriented class which is used for file handling in java.
- Unlike the FileOutputStream class, you don't need to convert the string into a byte array because it provides a method to write string directly.
public class FileWriter extends OutputStreamWriter
Program:
import java.io.FileWriter; public class FileWriterExample { public static void main(String args[]){ try{ FileWriter fw=new FileWriter("D:\\testout.txt"); fw.write("Welcome"); fw.close(); } catch(Exception e) { System.out.println(e); } System.out.println("Success..."); } }

Reading And Writing File In Java
Source: https://www.h2kinfosys.com/blog/reading-and-writing-text-file-java/
Posted by: goodmancrooking1973.blogspot.com
0 Response to "Reading And Writing File In Java"
Post a Comment